What Is Node JS?
Let us start this guide by understanding the foundational concept. So, Node.js is basically an open-source JavaScript runtime environment developed by Ryan Dahl in 2009. Since then, Node.js has become popular over time and is now became an integral part of many applications and websites used today.
In simpler terms, Node.js is a tool that allows developers to run JavaScript code outside of a web browser, Before Node.js, JavaScript was mainly used for creating interactive features in web browsers. However, with Node.js, developers can use JavaScript to handle backend development tasks like managing databases, handling requests from users, and even creating entire web servers. This makes Node.js a powerful and demanding development tool for building fast and efficient applications.
How Does A Node JS Work?
The working of Node Js is quite simple and easy. Let’s understand it clearly with the help of a practical example shown below-
Suppose you went to a food restaurant and placed an order at the counter, Now, instead of waiting there until your food is ready, you will simply get a receipt and move aside, allowing the next person in line to place their order. While you wait for your food to come, other customers whose foods are ready are being served, and once your food is ready, they will simply call your number, and you will pick it up.
Node.js works in a similar way. When a request is made to a Node.js server, Node.js doesn’t wait for that task to be completed. Instead of that, it handles other tasks while your request is being processed in the background. Once your task is completed, Node.js comes back to it and sends the response to you, telling you that it is successfully completed.
This way of working is known as non-blocking or asynchronous working and It allows Node.js to handle multiple tasks at the same time, This Asynchronous working makes it very efficient and fast, especially for applications that need to manage many requests at once, like websites or chat bots.
Why Do We Use Node JS?
There are plenty of reasons that makes node JS a preferrable choice among developers, some of the major reasons include-Â
- Being built on Google’s V8 engine, it is very quick and has less execution time as compared to others.
- One of the key reasons developers love Node.js is because of its rich collection of packages available in the Node Package Manager. With over 50,000 packages, Web developers can easily import the tools they want and save a lot of their time.
- Node.js is also great for building real-time, heavy-data web applications because it doesn’t have to wait for an API to send back data. This is due to its asynchronous nature, which means that it doesn’t block other operations while waiting for a response.
- Another advantage of using Node.js is that it helps to reduce the loading time for audio and video. This happens because the code is well-synchronized between the client and server and also allows them to use the same code base.
- Since Node.js is built on the JavaScript framework, it’s easy for developers who are already familiar with JavaScript to start using Node.js for their web development projects. This can significantly reduce the training time and cost of individual and company.
Features Of Node JS
Node.js has various unique features to offer that make it a standout choice in the web development market, some of its key features are written below for your better understanding of the concept-
1. Asynchronous and Event-Driven
Node.js is designed to handle tasks in a non-blocking way. This means it doesn’t wait for one task to finish before moving on to the next one. For example, when a Node.js server asks an API for data, it doesn’t sit idle while waiting for a response. In the meantime, it goes ahead to handle other tasks. Once the data from the API is ready, it again moves to that data to pick it up again. This makes Node.js fast and efficient because it doesn’t waste time waiting around.
2. Single-Threaded Architecture Â
Node.js uses a single thread to manage tasks, which makes it more scalable. While other servers that create multiple threads to handle requests, Node.js handles many tasks with just one thread through event looping. This allows Node.js to handle a large number of requests efficiently. Compared to traditional servers like Apache, Node.js can manage more requests at the same time.
3. Scalable and Efficient
Scalability is a big deal in software development, and Node.js excels in this area. It can efficiently handle multiple requests at once. Node.js uses a clustering module to balance the load across all available CPUs. It can also split applications into smaller parts, allowing for different versions of an app to be delivered to various users. This flexibility helps in customizing services to meet different client needs.
4. Fast Code ExecutionÂ
Node.js uses the V8 engine, the same engine that is used by Google Chrome. This engine makes code execution very fast. As a result, Node.js can handle requests quickly which makes it a speedy option for server-side programming.
5. Uses JavaScript
Node.js has its foundational base of JavaScript, a language that most of the developers are already familiar with. This makes it easy for developers to learn and work with Node.js.
6. Fast Data Streaming Â
Node.js can process and upload file at the same time, which speeds up the entire process. This quick handling of data streams makes Node.js a great choice for applications that require fast data and video streaming.
7. No Buffering
In Node.js, data is processed in chunks and is never buffered. This means data is handled more efficiently, with no delays, making the system faster overall.
Node JS Architecture
Now that we have understood the basics of Node JS, including its features, advantages and function. Let us move forward to one of the most important topic and understand its architecture. Here is a diagram provided by simform that best illustrates the architecture of the Node.Js, let us understand the step by step procedure with the help of this diagram-Â
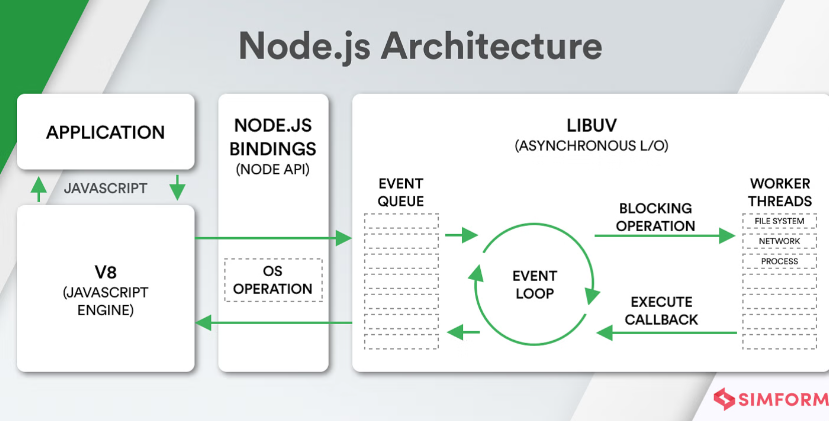
1. Application
At the top of the architecture, you can see the Application box, This box mainly contains the JavaScript code that you wrote to create your application. This code is the starting point where you define what your application should do.
2. V8 JavaScript Engine
The V8 box below the application box, mainly represents the V8 JavaScript engine. This is the engine that runs your JavaScript code. V8 is developed by Google and is also used in the Chrome browser. It converts your JavaScript code into machine code within seconds that your computer can understand and execute.
3. Connection to OS Operations
After the V8 engine processes your code, it’s time to perform operations that involve your computer’s operating system, like reading files or handling network requests. These are called “OS Operations.”
4. Node.js Bindings
The Node.js Binding box acts like a bridge between the JavaScript code you write and the operating system. This allows your JavaScript code to interact with the system’s hardware, like file systems or network interfaces.
4. Libuv (Asynchronous I/O)
Inside the Libuv box, there are various operations taking place. Let us understand each of them clearly-
- Event Queue: The Event Queue is where all the tasks that need to be processed are lined up. These tasks could be anything from reading a file, making a network request, or processing some data.
- Event Loop: The Event Loop is a key part of Node.js. It continuously checks the event queue to see if there are any tasks that need to be processed. If there are, it picks them up and processes them.
- Blocking Operation & Worker Threads: Some tasks might take longer to complete, like reading a large file or making a network request. These are called “Blocking Operations.” Instead of making the whole application wait, Node.js sends these tasks to Worker Threads. These threads handle the tasks in the background, allowing the main event loop to keep running smoothly.
- Execute Callback: Once a task is completed by the worker threads, a “Callback” is executed. This means that the result of the task is processed and returned to the application.
Node JS ModulesÂ
Modules in Node.js are like tools or libraries that you can add to your application to use specific functions directly. Now, To include a module in your Node.js application, you have to use the `require()` function, and inside the parentheses, you have to put the name of the module you want to use. Below is the basic representation defining how you can add Modules to your application-
Method To Add Node JS Module |
const http = require(‘http’);
http.createServer(function (req, res) { Â Â Â Â res.writeHead(200, { ‘Content-Type’: ‘text/html’ }); Â Â Â Â res.write(‘Welcome to PW Skills!’); Â Â Â Â res.end(); }).listen(3000); |
Node.js comes with several built-in modules that offer basic features needed for building web applications. Here’s a list of some commonly used Node JS modules and their uses.
Node JS Modules | Uses |
http | creates an HTTP server using Classes, methods, and events in Node.js. |
Assert | set of assertion functions useful for testing. |
Fs | used for handling file I/O operations. |
Path | includes methods to deal with file paths. |
Process | provides information and control the current Node.js process. |
OS | provides information about the operating system. |
Querystring | utility used for parsing and formatting URL query strings. |
Util | Includes useful utility functions for developers |
URL | module provides utilities for URL resolution and parsing. |
Applications Of Node JSÂ
Node.js is a powerful tool used for building different types of applications due to its fast and efficient working technology. Here are some common ways people use Node.js:
- Web Servers: Node.js is great for creating web servers that are quick and can manage a lot of users at the same time.
- Real-Time Apps: It works really well for apps that need instant updates, like chat apps, online games, or tools where people work together in real-time.
- APIs and Microservices: Node.js is also used to build APIs and microservices that allow different parts of an application to communicate with each other easily.
- Single-Page Applications (SPAs): For apps that run in a single page and update content dynamically without reloading, Node.js handles the backend processing of these apps and makes the process smooth and fast.
- Streaming Apps: Because Node.js can handle data as soon as it comes in, it’s ideal for streaming apps, such as video streaming services like netflix.
Who Uses Node JS?
In today’s digital world, almost every company wants their application to be fast and efficient so that they can handle multiple user queries at the same time.Â
Some of the top companies using Node JS in their daily task include –Â
NASA, Netflix, Trello, Paypal, LinkedIn, Uber, TwitterX, Flipkart, Amazon, and more.Â
Demand For Node JS
According to the recent Radixweb report, Over 6.3 million websites are estimated to use Node.js. This shows the importance of this language in today’s time.
One more report by esparkinfo, shows that by the end of 2024, the global number of Node.js developers is expected to reach 27 million in the market. This shows the rise in demand for skilled Node JS developers in the tech field. People with skilled Node JS and other relevant skills are more likely to earn higher salaries and work for Big MNCs.Â
Learn NodeJS With PW Skills
Start your career in the field of web development with our PW Skills Full Stack Web Development Course.Â
Enrolling in this course will provide you with 150+ hours of instructor-led live classes in a beginner-friendly manner. Apart from this, features like- daily assignments, regular doubt classes, PWSkills Lab for coding, 100% placement assistance, and course completion certificates will help you to stand out from the crowd.
Don’t waste your time, Visit PWSkills.com to book your seat today in this exciting journey.
DOM in JavaScript FAQs
How does Node.js handle concurrency?
Node.js handles concurrency using a single-threaded event loop model. Instead of creating multiple threads for handling concurrent tasks, Node.js process them in a non-blocking manner through callbacks, promises, and async/await patterns.
What are some common challenges when working with Node.js?
Common challenges include:
Callback Hell: Managing nested callbacks can become complex and hard-to-maintain.
Error Handling: Properly handling errors can be tricky in asynchronous code.
How do I start learning Node.js?
To start learning Node.js:
Begin with understanding JavaScript and asynchronous programming.
Set up Node.js on your computer and experiment with basic server creation.
Explore resources like articles, online tutorials, and PW Skills courses.
Practice by building simple applications, such as a web server or API.