Python Range function can help you iterate a block of code a specified number of times. The range function can accept a maximum of three parameters i,e. Start, end and step. In this blog, we will learn everything about the Python Range() function and learn how to use this built-in function with the Python programming language.
What Is Python Range Function?
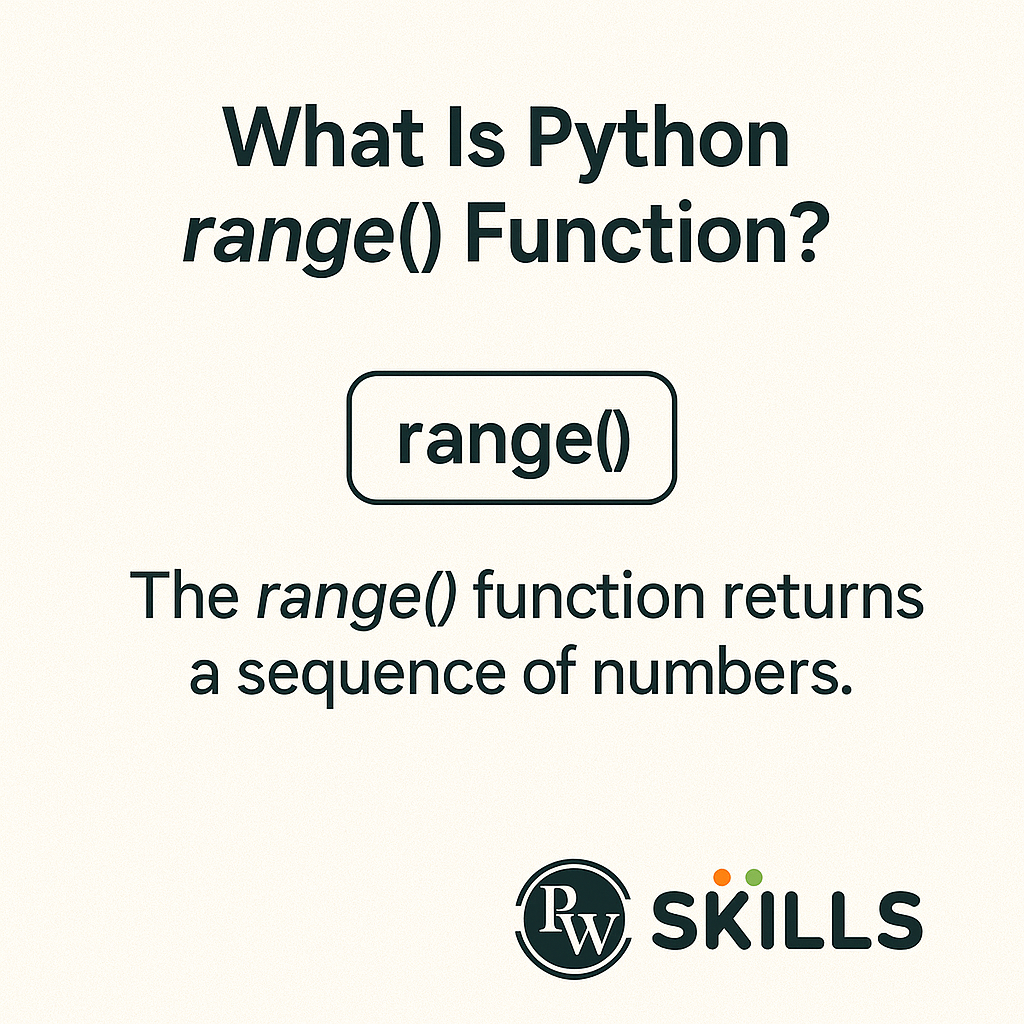
Python Range function is used to provide a range to generate a collection of numbers which can be iterated. The range( ) function can be used to apply the range method in Python. You can specify where to start, where to end and the number of steps you will take during the iteration.
The Python range function can have at most three parameters which can be used while working with for loops.
Python Range Function Key Takeaways
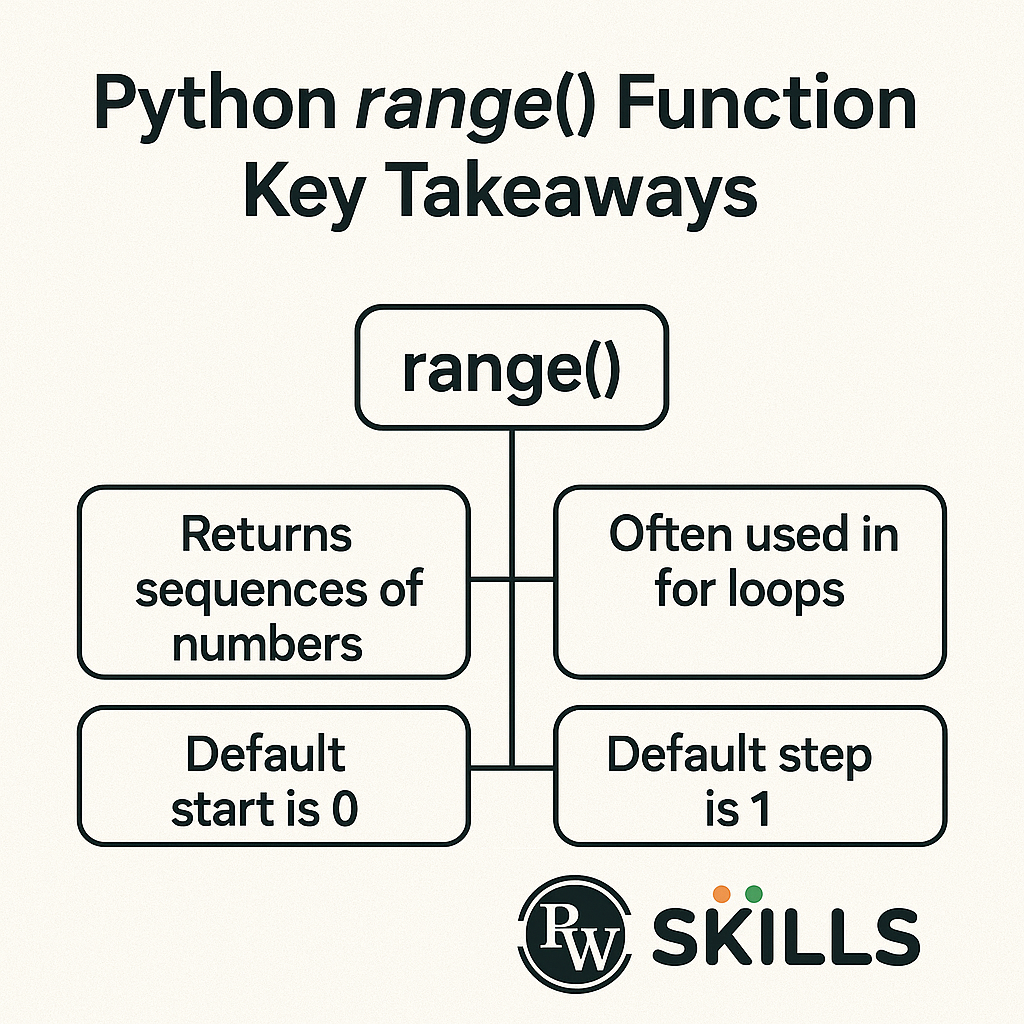
- Python Range Function can be used to iterate through a defined number of blocks for a specified number of times.
- The stop parameter is mandatory to provide in the range() function.
- The Python Range() function can start with a specified number and keep on incrementing by the defined number.
- The Range function in Python only works with the integers. We cannot use float or other data types with the range() function.
- The range() function is not inclusive.
- You can easily take positive and negative steps with the range function.
What Does Range Function Do In Python?
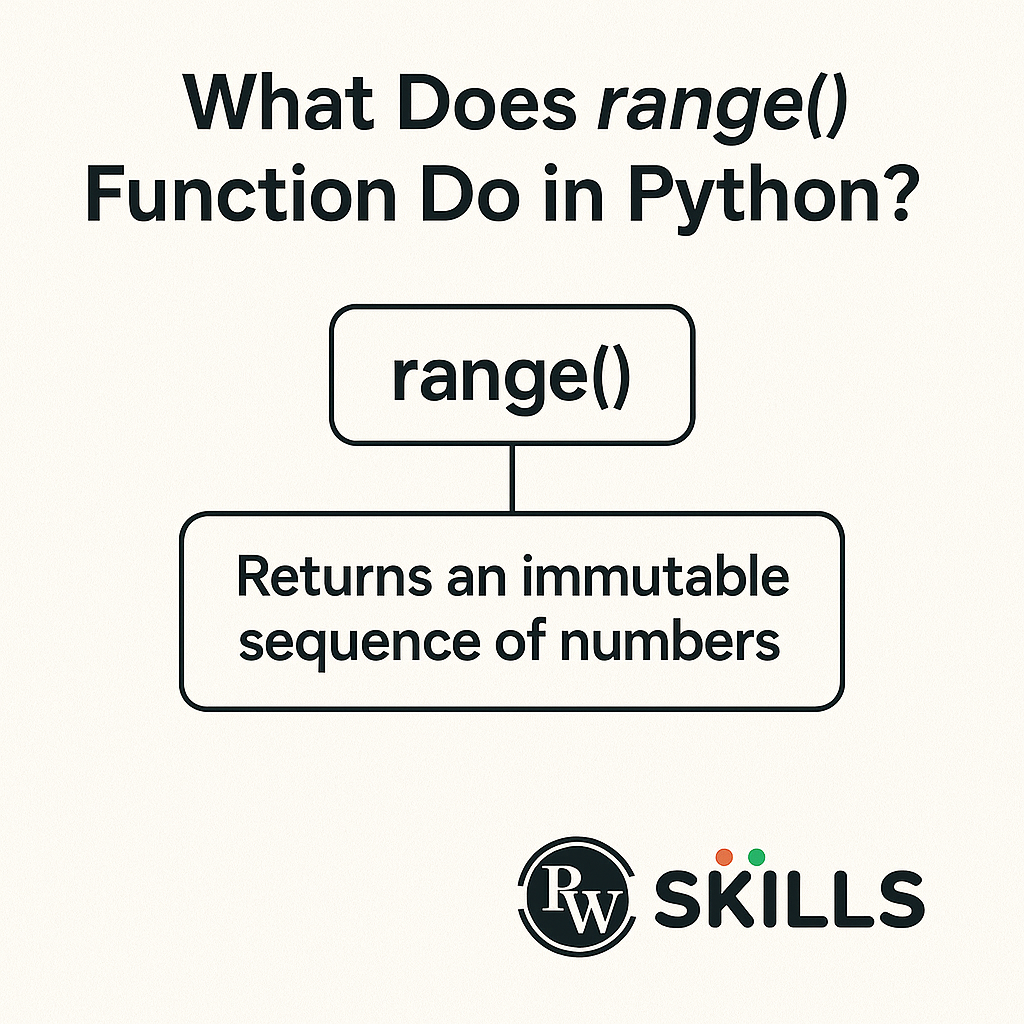
The Range Function is a built-in method in Python which can be used to return a sequence of numbers having three operational parameters as an argument inside.
Some of the defaults given in the Python range function are:
- The starting point in the Python Range function is always 0 unless you specify a custom value.
- The range() function always takes one step ahead as an increment.
- The Python Range function does not include the last given number i,e. Stop argument in the specified range.
Read More: Top Python Internships to Apply In April 2025
Examples of Using the Range() Function In Python
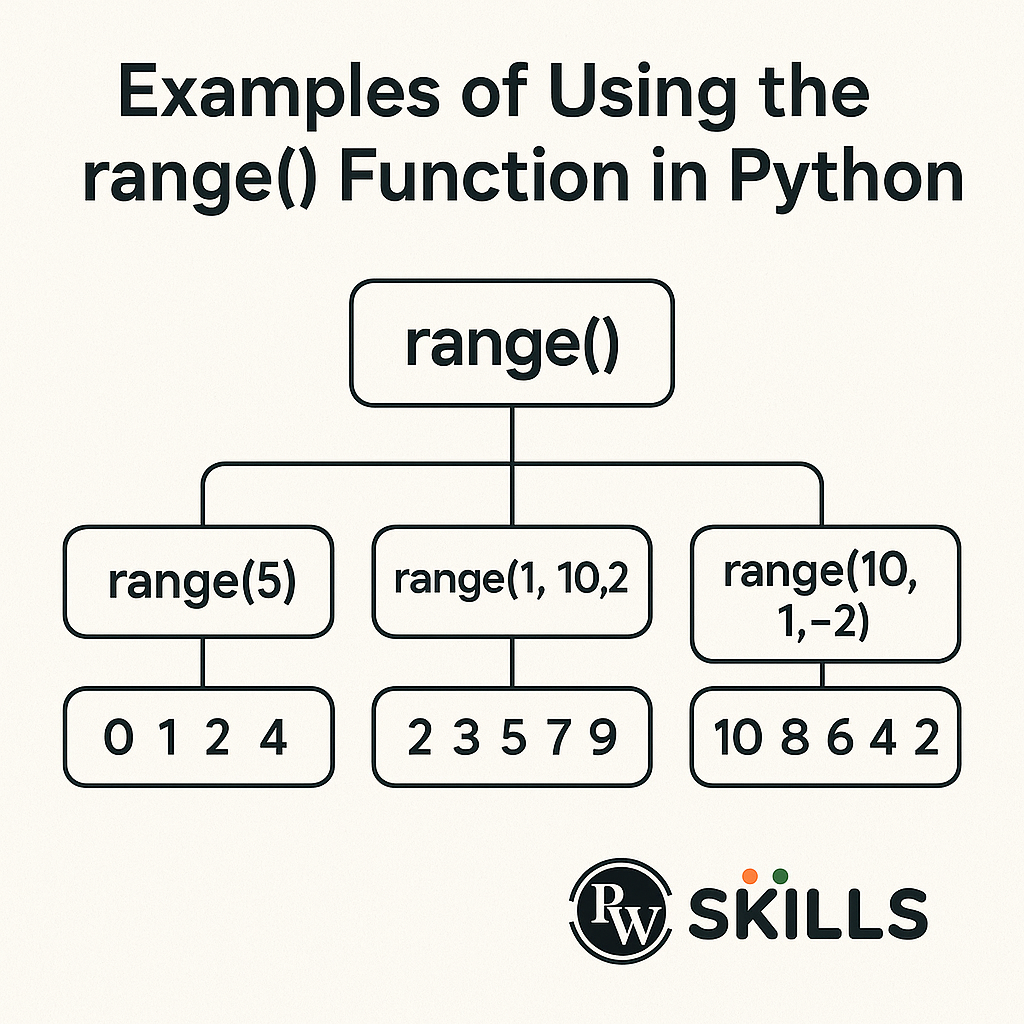
Let us look at some of the examples of using the range() function in Python.
1. Write a program to iterate in a list using the index values and print the value along with their index value.
fruits = [‘apple’, ‘banana’, ‘cherry’]
for i in range(len(fruits)): print(i, fruits[i]) |
Output
![]() |
2. Write a program to demonstrate reverse counting with Python range function.
for i in range(5, 0, -1):
print(i) |
Output
![]() |
3. Write a Python program to convert the range () into a list.
nums = list(range(5))
print(nums) |
Output
![]() |
Parameters of Python Range() Function
In Python Range function we can use a maximum of three parameters at a time. The built-in range function can be used with loops and move through the block of code for a specified number of times.
range (start, stop, step) |
Out of the three parameters in Python Range() function one is mandatory and other two are optional in Python.
- The Stop argument is mandatory in range() function in Python.
- The range function can start from a given number i,e. Start and end with the value specified on the position i,e. Stop.
- When you do not want to start iterating from 0 then you can specify the starting point using the “Start” tag.
- The “start” argument is optional where you can specify a starting value.
- Also, if you do not want to increment each value by 1 then you can specify a third optional argument i,e. Step.
- With steps you can make increments according to your need until you reach the stop argument.
How to Use the Range() Function With Start and Stop Arguments?
You can easily use the start and stop function as a parameter in range() function. It is important to note that the start argument in the Python range() function is inclusive while the stop argument is not inclusive. For example, if you want to print a number in the range from 4 to 10 then you will have to take the range ( 4, 11) to get numbers printed from 4 to 10.
for num in range (4,11):
print (num) |
Output
![]() |
This condition is valid also when you use a negative value in the range function. The stop argument in the range() function is exclusive and does not include the number itself. For example, let us take a range of (-7, 2) then you will get output as
for num in range (-7,2):
print (num) |
Output
![]() |
Hence, it is very much important to be careful while defining the Python range function.
How to Use the Python Range Function With All Three Parameters?
You can use all three parameters in Python range function at a time. By default the “step” argument in Python is 1 but you can change the increment or decrement step value based on your need.
Suppose we want to take 2 step every time, then we can keep the value of step as 2 and run the range function.
for num in range (9, 15, 2):
print (num) |
Output
![]() |
You can see the output result takes 2 steps every time it iterates and prints the number in the range of 9 to 15. The value 15 is not taken into consideration as the “stop” argument is not inclusive.
Can We Use The len() Function With Python Range Function?
We can easily take use of the len() function in Python to define the range of a list, tuple, or other data storage containers. The len() function helps us get the desired range for which we need to print the values. The value stored after the len() function return can be used as an argument in the Python Range () function.
cars = [ “Toyota”, “Maruti”, “Tata”, “Mahindra”, “Volvo”, “BMW”]
len_cars = len(cars) for car in range(len_cars): print(“Got my Car”) |
Output
![]() |
Can We Decrement Using the Python Range Function?
Yes, we can take negative steps using the range() function. To make the reverse step make sure your steps have negative value and it is decrementing not incrementing.
Let us understand it with the help of a simple example, suppose the range you have is from 15 to 0 and you have to decrement -1 every step of the way.
for num in range(15,0,-1):
print (num) |
Output
![]() |
Benefits of Using Python Range Function
Let us know some of the major benefits of using the Python range() function below.
- The range() function returns a range object and does not store all numbers in memory and hence it makes it efficient.
- With range function looping, implementation becomes easy to repeat a block of code a certain number of times.
- It provides flexible parameters which can be used in the function whenever needed such as start, stop and step.
- The Python range function works well with the len() method in Python.
- You can easily convert the return values of the range function into a list.
- It supports negative steps and custom increments based on your input.
The above benefits make Python range() function an optimal choice as it is lightweight, no overheads, versatile, and make clean and readable loops.
Learn Data Analytics with PW Skills
Become proficient in data analysis and extract useful insights from data using various techniques, tools, and methods. Get in-depth tutorials, hands on learning, practice exercises, and module assignments throughout this Data Analyst Course offered on PW Skills.
Learn from dedicated mentors and master in tools like Excel, SQL, Python, PowerBI, etc. Get certification from PW Skills after completing the course.
Python Range Function FAQs
Q1. What is Python Range Function?
Ans: Python Range function is used to provide a range to generate a collection of numbers which can be iterated. The range( ) function can be used to apply the range method in Python.
Q2. What are the parameters in Python Range Function?
Ans: Start, stop, and step are the three parameters in Python Range() function.
Q3. Is range() function present by default in Python?
Ans: The range() function is an in-built function in Python which is already defined and we can use it to return a sequence of numbers using loops.
Q4. Which parameter in the Python range function is an option?
Ans: In Python range function, start and step are the optional arguments while stop is a mandatory argument.