In today’s tech-dominated world, Python is one of the most popular programming languages used in a variety of tasks. While it’s a general-purpose language, it’s used in many different fields like Machine Learning, Artificial Intelligence, web development, IoT, and more.
This Py tutorial is specially designed for beginners to help them learn the basics of python programming language and gradually move to more advanced python topics. By the end of this Py tutorial, you’ll have a strong understanding of Python, and you’ll be ready to continue learning and become a top-level Software Engineer.
What Is A Python Programming Language?
Python is a widely used programming language known for being flexibility and easy-to-understand syntax. It is an interpreted language, which means you can run Python code without needing to compile it first. Apart from this, Python is also an object-oriented and high-level language which makes it easier to read and write even for beginners.
One of Python’s main strengths is that it supports different programming styles, including procedural – which focuses on writing sequences of instructions, object-oriented – which focuses on objects and classes, and functional programming – which focuses on using functions.
Python was designed by Guido Van Rossum with a focus on enhanced readability, so the code is usually easy to understand with clear syntax and properly organized code blocks. This makes Python a great choice for both beginners and experienced programmers.
Demand For Python
Python is one of the most in-demand programming languages today, and major companies are actively looking for skilled Python Developer to develop their websites, software, and applications, as well as helping them in working with Data Science, Artificial Intelligence (AI), and Machine Learning (ML) technologies.
According to a recent Glassdoor survey, A Python Programmer with 3-5 years of experience can earn around 8 – 10 Lakh per annum in India. Which makes it one of the most favorite and demanding programming languages. However, salaries can vary depending on the job location and company. Some of the big companies that use Python programming language and recruit a high number of Python developers include:
Characteristics Of Python Programming Language
You must be wondering why this language is so favorite of developers, Don’t worry here are some important features of Python programming languages that will help you to clear all your doubts:
- Python allows multiple programming styles, like functional, structured, and object-oriented programming (OOP).
- You can use Python for scripting small tasks or compile it into byte-code to build larger applications.
- It offers very flexible data types and checks data types automatically as the program runs.
- Python handles memory management on its own, so you don’t have to worry about cleaning up unused data.
- You can easily combine Python with other languages like C, C++, COM, ActiveX, CORBA, and Java.
Features Of Python
Python is a powerful and popular programming language known for its many features. It’s a high-level, interpreted language which means it reads and executes code line by line. Let’s explore some of the key features that make Python stand out language in the market.
1. Easy to Learn
One of the main reasons people love Python is because it’s easy to learn. The language uses simple syntax, where the rules and structure are straightforward. It also uses indentation instead of curly brackets which makes code cleaner and easier to read. Python doesn’t require you to declare variable types beforehand, which makes it beginner-friendly.
2. Dynamically Typed
Python is dynamically typed, which means you don’t need to specify the type of variable when you create it. The type is determined at runtime, depending on the value you assign to it. This makes coding in Python more flexible and easier, especially for beginners.
3. Interpreter-Based
Python is an interpreter-based language. This means it reads and executes your code line by line. If there’s an error in one part of your code, Python will run the code up until that point. This feature makes it easier to find and fix mistakes, which is great for those new to programming.
4. Interactive Mode
Python comes with an interactive mode that allows you to test small bits of code quickly. You type in a command, and Python will immediately give you the result. This is especially useful for learning and experimenting with new ideas.
5. Multi-Paradigm
Python is not just object-oriented; it also supports other programming styles like procedural and functional programming. This means you can choose the style that best suits your project, making Python very flexible.
6. Rich Standard Library
Python has a large standard library that comes with many useful modules and packages. These tools make it easier to handle tasks like data processing, internet communication, and more without needing to write everything from scratch.
7. Open Source and Cross-Platform
Python is open source programming language which means you can download and use it for free. It works on various operating systems like Windows, Linux, and MacOS, making it a cross-platform language. You can write Python code on one system and run it on another without any changes.
8. GUI Applications
Python can be used to create graphical user interface (GUI) applications. It comes with a library called Tkinter for building GUIs, and other popular tools like PyQt and WxWidgets are available to create user-friendly applications.
9. Database Connectivity
It can be connected to almost any type of database. Whether you’re working with traditional databases like MySQL or modern NoSQL databases like MongoDB, Python has all the tools you need.
10. Active Community
Python has a large and active community of developers who contribute to forums, conferences, and the ongoing improvement of the language. This strong support network makes learning Python easier and more enjoyable.
Steps For Python Download
Before starting the installation process of Python- please note that python version 3.10 and newer cannot be installed on Windows 7 or earlier versions. Here’s a simple steps written below that will help you in installing Python on Windows:
- Step 1- Go to the official Python website to choose the latest stable version and download the 64-bit installer.
- Step 2- Locate the downloaded file in your computer and double-click it to start the installation process.
- Step 3- You can click “Install Now” to begin the installation, but it’s better to customize the settings. Choose a folder with a short path for installation and also check the box that says “Add Python to PATH.”
- Step 4- Accept the default settings and continue with the installation.
- Step 5- After the installation process is completed, open the Command Prompt on your PC. Type `python` there and press Enter to make sure Python is installed correctly. If the installer responds with the version installed on your computer. Congratulations, you’ve successfully installed Python on your Windows computer.
Python Syntax
Python syntax is a set of rules that you follow to write a Python program. It tells you how to structure your code so that Python can understand it. Python’s syntax is similar to other programming languages like Perl, C, and Java, but it is considered much more easy to read and write compared to them. Let’s look at a basic example to understand Python syntax better. Here’s a simple Python program that prints a message:
Py Tutorial – Python Syntax Example |
print(“Hello, world!”) |
Output-
Hello, world! |
In this example shown above:
- `print` is a function that tells the compiler to print the text on the screen.
- “Hello, world!” written inside quotation marks is the message that we want to display.
Variables In Python
Python variables are like storage boxes in your computer’s memory where you can keep different types of data, such as numbers or letters, while working on a Python program. When you create a variable, you are basically reserving some space in the computer’s memory to hold that data.
The type of data you put in the variable, like an integer, a decimal value, or a character, helps Python to decide how much space it needs in the memory and what kind of data can be stored there.
How To Create Python Variables:
You don’t need to do anything special to create a Python variable; it creates automatically when you assign a value to it. You basically use the equal to (=) sign to do this. Let us understand this with the help of an example
Py Tutorial – Python Variables Examples |
Marks = 100 # Creates an integer variable
Pie = 3.17 # Creates a floating point variable Name = “PW Skills” # Creates a string variable |
When you do this, the variable is created automatically and the value is stored in it. This makes working with variables in Python very easy and beginner-friendly.
Data Types In Python
Data types in Python are like blueprints, and the variables we create are based on these blueprints. Since Python is dynamically typed, the type of data a variable holds is decided while the program is running, depending on the value assigned to it. Data types help us to understand what kind of data a variable can hold and what actions we can perform on that data. Here is a simple flowchart written below which will help you to understand different types of Data types in Python more clearly.
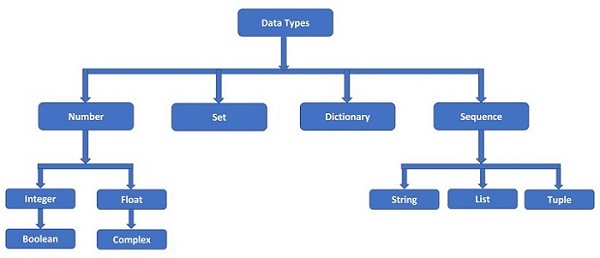
1. Numeric Data Type
In Python, numeric data types are used to store different types of numbers. When you assign a value in the form of number, a number object is created. Python has four main types of numeric data types, each with its own class in the Python library: int, float, bool, and complex.
- Int: This type is used for storing whole numbers. They can be positive or negative, like 3 or -5.
- Float: This type is used for storing numbers with decimal points, also called floating-point numbers. For example, 3.14 or -0.001.
- Complex: This type is used for storing complex numbers, which have two parts: a real part and an imaginary part. In Python, a complex number is written as `x + yj`, where `x` is the real part, and `y` is the imaginary part. The imaginary part is always followed by the letter `j`.
2. Sequence Data Types
In Python, sequence data types are a way to store and organize collections of items in an ordered manner. Each item in a sequence has a specific position, starting with 0. This is similar to arrays in languages like C or C++. Python has three main sequence data types:
1. Python String Data Type:
- A Python string is a sequence of one or more characters which can include- letters, numbers, symbols enclosed in single, double, or triple quotes.
- Strings in Python are immutable, which means once you create a string, you can’t change it. If you perform any operation on a string, it creates a new string instead of modifying the original one.
Py Tutorial – Python String Data Type Example |
str = ‘Welcome To PW Skills!’
print (str) # Prints complete string print (str[0]) # Prints first character of the string print (str[2:5]) # Prints characters starting from 3rd to 5th print (str[2:]) # Prints string starting from 3rd character print (str * 2) # Prints string two times print (str + “TEST”) # Prints concatenated string |
Output-
Welcome To PW Skills! W lco lcome To PW Skills! Welcome To PW Skills!Welcome To PW Skills! Welcome To PW Skills!TEST |
2. Python List Data Type:
- Python lists are one of the most flexible data types in Python. A list is a collection of items separated by commas and enclosed in square brackets `[ ]`.
- Unlike arrays in C, Python lists can hold items of different data types, such as numbers, strings, tuples, dictionaries, sets, or even objects from user-defined classes.
- Lists are mutable which means you can change their elements or size after they are created. This is different from tuples, which are also sequences but are immutable and enclosed in parentheses `()`.
Py Tutorial – Example Of List Data Type In Python |
list = [ ‘pwskills’, 786 , 3.14, ‘Py Tutorial’, 99.9 ]
tinylist = [798, ‘sahil’] print (list) # Prints complete list print (list[0]) # Prints first element of the list print (list[1:3]) # Prints elements starting from 2nd till 3rd print (list[2:]) # Prints elements starting from 3rd element print (tinylist * 2) # Prints list two times print (list + tinylist) # Prints concatenated lists |
Output-
[‘pwskills’, 786, 3.14, ‘Py Tutorial’, 99.9] pwskills [786, 3.14] [3.14, ‘Py Tutorial’, 99.9] [798, ‘Py Tutorial’, 798, ‘Py Tutorial’] [‘pwskills’, 786, 3.14, ‘Py Tutorial’, 99.9, 798, ‘Py Tutorial’] |
3. Python Tuple Data Type:
- Tuples are similar to lists but have one key difference—they are immutable. Once you create a tuple, you cannot change its elements or size.
- Tuples are enclosed in parentheses `()`, making them a good choice for storing data that shouldn’t be changed. You can think of tuples as read-only lists.
Py Tutorial – Example Of Tuples Data Type In Python |
tuple = ( ‘pwskills’, 786 , 3.14, ‘sahil’, 99.9 )
tinytuple = (798, ‘sahil’) print (tuple) # Prints the complete tuple print (tuple[0]) # Prints first element of the tuple print (tuple[1:3]) # Prints elements of the tuple starting from 2nd till 3rd print (tuple[2:]) # Prints elements of the tuple starting from 3rd element print (tinytuple * 2) # Prints the contents of the tuple twice print (tuple + tinytuple) # Prints concatenated tuples |
Output-
(‘pwskills’, 786, 3.14, ‘sahil’, 99.9) pwskills (786, 3.14) (3.14, ‘sahil’, 99.9) (798, ‘sahil’, 798, ‘sahil’) (‘pwskills’, 786, 3.14, ‘sahil’, 99.9, 798, ‘sahil’) |
Operators In Python
Operators in python are special symbols that help you to perform specific actions on variables, values, or expressions. For example, the addition operator `+` in Python is used to add two numbers or variables together. Here are some key terms related to Python operators that will help you to understand the concept in a better way:
- Unary Operators: These operators need only one value to do their job. For example, the `-` sign can be used to make a number negative.
- Binary Operators: These operators need two values to work. For example, the `+` operator will add two numbers together.
- Operands: These are the values, variables, or expressions on which the operators act upon.
Types of Python Operators
Python has different types of operators where each one is used for a specific purpose, some of the commonly used operators in python are explained blow for your reference
- Arithmetic Operators: These operators are used for basic math operations like addition, subtraction, multiplication, and division.
- Comparison Operators: These are used for comparing two values and return `True` or `False` based on that. For example, `>` checks if one value is greater than another.
- Assignment Operators: These are used for assigning a value to a variable. For example, `=` is used to assign a value like `x = 5`.
- Logical Operators: These are used to work on conditional statements. For example, `and`, `or`, and `not` are logical operators.
- Bitwise Operators: These work with the binary representation of numbers. For example, `&` is used for “AND” operations at the initial level.
- Membership Operators: These operators are used for checking if a value is part of a sequence or not. For example, `in` operator is used for checking if a value exists in a list.
Understanding these operators help you to perform different operations in Python and makes it easier for you to write programs that can handle a variety of tasks.
Learn Python With Our Python Course
Are you looking to become a skilled Python programmer but feel confused by all the courses out there? Don’t worry, we’re here to help! Enroll in our PW Skills Python with DSA course to learn everything you need to know about Python programming with the essence of DSA.
This beginner-friendly course is of 6 months and starts with the basics of Python. As you progress, you’ll move on to more advanced topics, helping you to become a confident and proficient Python programmer. What makes this course a demanding and favorite choice of students is its in-demand industry-relevant curriculum, guidance from expert mentors, hands-on practical projects, networking opportunities, access to skills lab for code practice.
Visit PWSkills.com today to sign up and take advantage of exciting discounts on course fees!
Py Tutorial FAQs
How do I start learning Python as a beginner?
To learn Python start with the basics first, such as understanding variables, data types, and control structures like loops and conditional statements. Consider online tutorials like PWskills, coding platforms, and Python documentation to practice writing simple scripts.
What are some good resources for learning Python?
There are many free and paid resources available on the internet to learn python but talking about the best one- PW skills is the one stop destination for all your needs which also provides access to its lab for coding practice.
What types of projects can I build with Python as a beginner?
Start with small projects like a calculator, to-do list, or a simple game. As you progress, you can build web apps using Flask or Django, data analysis projects, or even simple automation scripts.