C++ is a must-know programming language that has been a favorite among coders for many years. Even though it was created in the mid-80s, it’s still as important today as it was back then. C++ is a general-purpose and object-oriented language, widely used in coding for various applications. Because of its reliability and performance, many top companies like LinkedIn, Microsoft, Opera, NASA, and Meta use C++.
If you want to work at these companies, it’s important to be familiar with the top C++ interview questions. So, to help you in preparing for your C++ interview questions we’ve gathered the top 20 most asked C++ interview questions for candidates. Going through these most asked C++ interview questions can help you in impressing recruiters and increase your chances of getting hired at top MNCs.
1. What is C++? What are the advantages of C++?
Ans) C++ is a programming language that was created to improve the older C language. It uses something called object-oriented programming (OOP), which means it organizes data into objects. These objects use concepts like polymorphism, inheritance, abstraction, encapsulation, and classes for a better and smooth programming experience.
Advantages of C++:
- C++ is a language that supports multiple programming styles, allowing you to structure your code in different ways.
- It also allows for dynamic memory allocation, which means it gives you control over how memory should be used in your programs.
- As a mid-level programming language, C++ can be used to create a variety of applications ranging from games development to desktop software to system drivers.
2. What are the different data types present in C++?
Ans) In C++, data types are used to specify the type of data that a variable can hold. There are three classifications of data types: primary, derived, and user-defined. all these are further derived into various data types each used for a different purpose. Let us look at the diagram below to understand what each data type has:
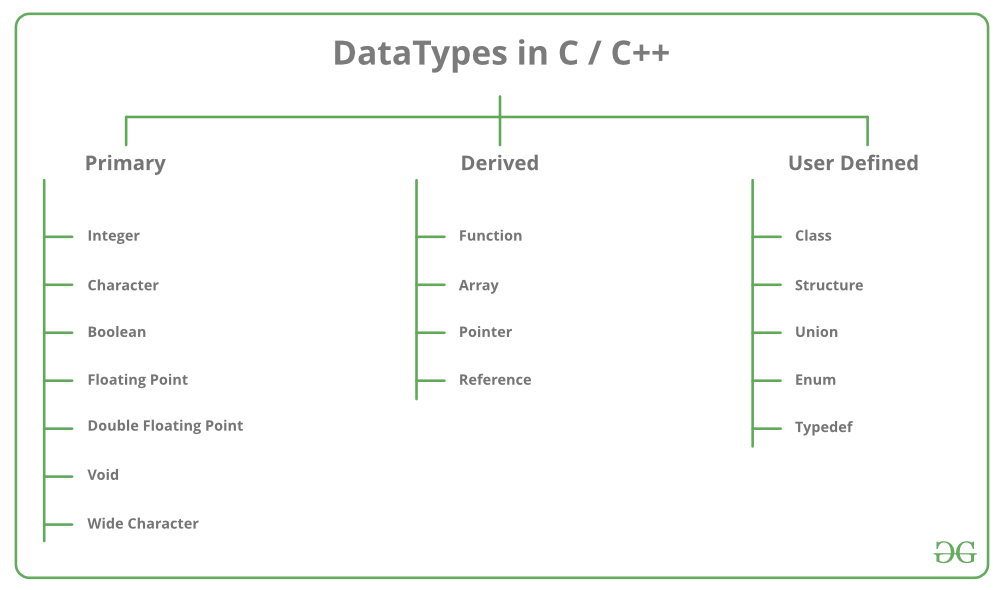
3. What is ‘std’?
Ans) ‘std’ stands for standard and is a namespace in C++. A namespace is like a container that holds various functions and objects. You must have wondered why every programmer writes “using namespace std;” before starting the program, it is because they are telling the compiler to use everything inside the std namespace. This allows them to use common functions like “cout” and “cin” without having to write “std::” every time before them.
4. What are references in C++?
Ans) In C++, a reference is like a nickname for an existing variable. When you create a reference, you are making a new name for the original variable. Any changes made to the reference also affect the original variable. To create a reference in c++ you generally use the ‘&’ symbol before the variable name.
5. Define Token In C?
Ans) In C++, a token is the smallest part of a program that the compiler can recognize and understand. Tokens are like the building blocks of the code which include various types like:
- Keywords: These are special words that have a fixed meaning in the language, like `int`, `return`, etc.
- Identifiers: Identifiers are the name given to things like variables or functions to identify them.
- Constants: These are values that do not change during the execution of the program, like numbers or fixed text.
- Strings: A sequence of characters, like a word or sentence, usually enclosed in double quotes.
- Special Symbols: A character with specific purposes in the code, like brackets `[]`, parentheses `()`, braces `{}`, semicolons `;`, etc.
- Operators: Symbols that perform operations on data, like `+`, `-`, `*`, `=`, etc.
These tokens are the basic elements that help in framing up your program and allow the compiler to understand what you want the program to do.
Q6) What is the difference between C and C++?
Ans) C++ is nothing but just an advanced version of C programming language which contains all the features of C programming language with some extra unique features. Let us understand the difference between both of them through the table given below.
Difference Between C And C++ | |
C | C++ |
It is a procedural programming language. | C++ is an advanced version of C that contains procedural and object-oriented programming languages. |
It does not support concepts like polymorphism, data abstraction, encapsulation, classes, and objects. | It supports all OOPs concepts and make programming much easier. |
It does not support Function and Operator Overloading | C++ supports function and Operator Overloading. |
7. What is the difference between struct and class?
Ans) Let us understand the difference between struct and class with the help of the table given below-
Struct | Class |
Struct members are always in a public mode by default. | Class members can be in public, private, and protected modes. |
Structures only hold value in memory. | Classes hold a reference of an object in memory. |
The memory in this case is stored as stacks | The memory here is stored as heaps. |
8. What is the difference between a reference and a pointer?
Ans) Difference between reference and pointer is an important C++ interview questions often asked in many technical interviews. Let us understand their difference with the help of table given below.
Reference Vs Pointer | |
Reference | Pointer |
The reference value can not be reassigned | The pointer value can be reassigned |
Reference can never hold a null value | The pointer can hold a null value, which is known as a null pointer |
Reference generally cannot work with arrays | The pointer can work well with arrays |
The location of the memory here can be accessed easily. | The pointer memory location cannot be accessed easily. |
9. Explain the difference between Array and List?
Ans) Array and list are two different things in a programming language which often configure beginners, let us understand the clear difference between them with the help of an example.
Arrays | Lists |
Arrays store elements of the same data type in consecutive memory locations. | Lists are collections of elements that can be of different data types, linked together with pointers. |
Arrays have a fixed size, meaning the number of elements cannot change after the array is created. | Lists are dynamic, so you can add or remove elements, changing the size as needed. |
Arrays use less memory because they only store the elements. | Lists use more memory because they store both the elements and the pointers linking them. |
10. What Is the difference between while loop and Do-while loop
Ans) While loop and do-while loop may sound a similar term but they are actually quite different from each other and both of them are used for different purposes. Let us look at the table below to understand the difference btween these two.
While Loop | Do-While Loop |
The while loop is known as an entry-controlled loop because it checks the condition before running the loop’s statements. | The do-while loop is called an exit-controlled loop because it runs the loop’s statements at least once before checking the condition. |
If the condition is false at the start, the loop’s statements will not execute at all. | Even if the condition is false, the loop’s statements will execute at least once. |
Example:
while(condition) { // statements } |
Example:
do { // statements } while(condition); |
11. What are Classes and Objects in C++?
Class: A class is like a blueprint that defines a new data type, where you can create objects that have particular attributes and functions. For example, if you have a `Car` class, it may include attributes like `color` and `model` and functions like `drive()` or `brake()`.
Object: An object on the other hand is a subset of a class. If `Car` is the class, then your particular car with a certain color and model is an object of that class.
12 What is Function Overriding?
Function Overriding occurs when a function in a derived class has the same name, parameters, and return type as a function in its base class. When this happens, the function in the derived class is used instead of the one in the base class. This is a feature of Runtime Polymorphism or Late Binding, where the correct function is called during the program’s execution.
13. What is Inheritance?
Ans) Inheritance is an important property in OOPs. when the child class can use properties and functions from the parent class it is known to be an inheritance. It’s like getting traits from your parents just like you might have your dad’s eyes or your mom’s smile, a child class can inherit features from a parent class. This allows you to reuse and extend existing classes without changing them.
14. What is Multiple Inheritance?
Ans) Multiple inheritance is when a child class can inherit properties and functions from more than one parent class. This is helpful when the child class needs features from different classes. For example, relate it with your real life by considering of your parents – Parent A (your dad) and Parent B (your mom). You being their child will inherit traits from both of them. Similarly, a class can inherit from two or more classes.
15. What is Polymorphism in C++?
Ans) Polymorphism generally means “many forms.” In C++, it refers to the ability of functions or operators to work in different ways depending on the type of data they are given. For example, just like a person can play different roles of a father, an employee, brother, or a friend. polymorphism also similarly allows the same function to behave differently depending on which object is calling it.
There are two types of polymorphism:
- Compile-Time Polymorphism
- Run-Time Polymorphism
16. What is a Constructor in C++?
Ans) A constructor is a special function in a class that has the same name as that of the class. Its main job is to initialize objects of that class. When you create an object, the constructor sets up the object with initial values.
There are three types of constructors that are primarily used in C++:
- Default Constructor: It takes no arguments and is called automatically when an object is created.
- Parameterized Constructor: It takes arguments and is called explicitly when values need to be passed to set up the object.
- Copy Constructor: Creates a new object by copying the values from an existing object of the same class.
17. What is a Destructor in C++?
Ans) A destructor is a special function in a class that cleans up the object when it is no longer needed. It has the same name as that of the class but is preceded by a tilde (~) sign. When the object goes out of scope, the destructor automatically deletes the object’s data and frees up memory.
18. How Are Delete[] and Delete functions different from each other?
Both, Delete[] and Delete functions have different roles to play in the programming language. Let us understand the major difference between them with the help of a table given below.
Delete Vs Delete | |
Delete | Delete[] |
Frees memory allocated for a single object | Frees memory allocated for an array of objects |
It is used when memory is allocated using new | It is used when memory is allocated using new[] |
The syntax used in this includes-
delete pointer; |
The syntax used in this includes-
delete[] pointer; |
It deallocates memory for a single object | It deallocates memory for all elements in the array |
19) What are C++ Access Modifiers?
Ans) Access modifiers in C++ are keywords that are used to set the level of access for members inside a class. Using these modifiers determine who can see or use these members and who cannot.
There are three main types of access modifiers that are mainly used:
- Private:
- Members declared as private can only be accessed or viewed within the class itself.
- These members are hidden from the outside world.
- Protected:
- Members declared as protected can be accessed within the class and by derived classes.
- These members are not accessible from outside the class unless it’s a derived class.
- Public:
- Members declared as public can be accessed or viewed from anywhere, both inside and outside the class.
- These members are fully exposed to the outside world.
20. What is an Abstract Class and When Do You Use It?
Ans) An abstract class in C++ is a class that cannot be used for creating object directly. It’s like a blueprint that is meant to be used by other classes. Some of the key points defining the usage of abstract classes are written below for your reference:
- Contains Pure Virtual Functions: An abstract class has at least one pure virtual function, which means the function is declared but not defined in the abstract class.
- Used as a Base Class: You can use an abstract class when you want to create a base class that provides common functionality to all its derived classes.
- Cannot Instantiate Directly: You cannot create objects of an abstract class. But you can create pointers or references to it, and you can use it to declare methods that must be implemented in derived classes.
Learn C++ With PW Skills
Enroll in our detailed PW Skills C++ programming course which is thoroughly crafted by experts considering various needs and demands of the students.
This 6-month-long beginner friendly course comes with the key features like – mentorship from expert trainers, skills lab access for code practice, daily practice sessions, regular coding competitions, job placement assistance, networking opportunity, resume review by experts, and much more.
So what are you waiting for? Enroll today and start your journey of becoming a proficient programmer!
C++ Interview Questions FAQs
What is a virtual function in C++?
A virtual function is a function in a base class that you expect to be redefined in derived classes. It ensures the correct function is called based on the object’s type, even when using a pointer to the base class.
What are the basic concepts of Object-Oriented Programming (OOP) in C++?
OOP in C++ includes concepts like:
Class
Object
Inheritance
Polymorphism
Encapsulation
Abstraction
What is the difference between new and malloc?
New is a C++ operator that allocates memory and calls the constructor.
Whereas malloc on the other hand is a C function that allocates memory but does not call the constructor. new is generally preferred in C++.