The 30 Days Of Python course will help you level up your Python skills. Whether you’re new to programming or learning Python for your interview, PW Skills’ Python for AI course is the perfect way to master Python for machine learning and AI applications. Over 4 comprehensive weeks, you will gain in-demand abilities to manipulate data, visualize insights, and build predictive models.
Hands-on coding challenges in our online labs will give you practical experience with essential Python libraries like NumPy, Pandas, Matplotlib, and more. Our expert instructors break concepts down into bite-sized lessons so you can code along every step of the way.
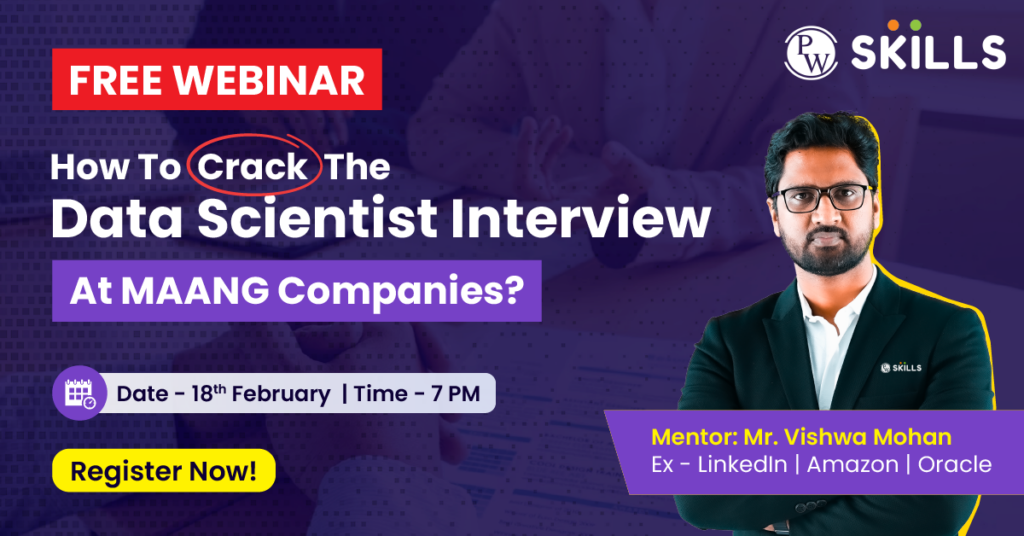
By the end of 30 Days Of Python, you’ll have multiple Python projects for your portfolio to showcase in job interviews. PW Skills supplements your learning with community support, notes, exercises, and mentorship.Â
Enroll now in this free course to elevate your Python coding skills for lucrative roles in AI and data science. The program starts February 18th, so reserve your seat today!
30 Days Of Python
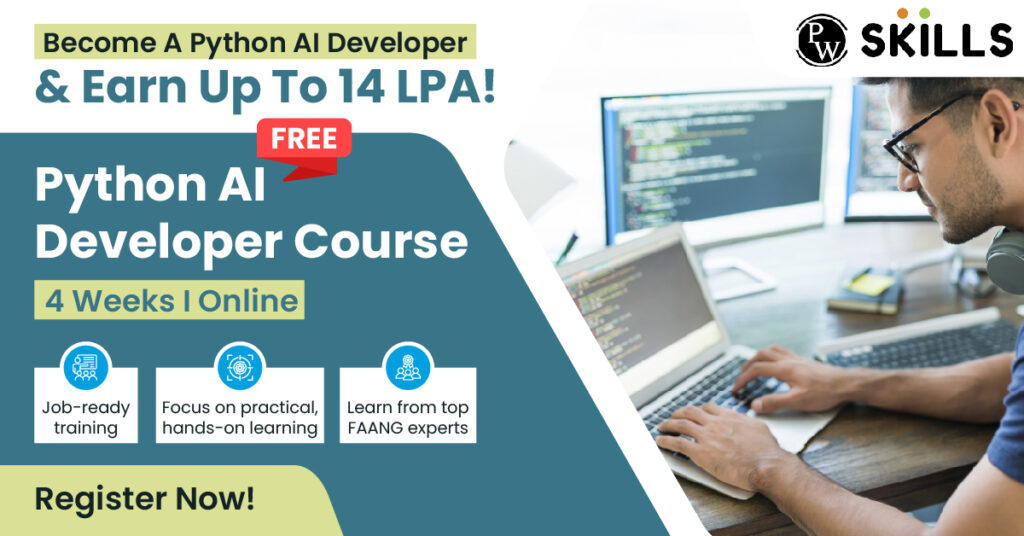
Starting on the 30 Days of Python journey is an excellent way to dive into the world of programming or enhance your existing skills. This structured approach ensures consistent practice, which is crucial for mastering any new skill, especially programming.Â
Through daily coding challenges and hands-on projects, participants will gradually build a strong foundation in Python programming.Â
Let’s delve into the modules covered in the 30 Days Of Python course:
Module 1: Python Basics
- Introduction to Python programming language
- Syntax, variables, data types, and basic operations
- Control flow statements: if, elif, else, loops
Module 2: String Objects
- Manipulating strings: slicing, concatenation, formatting
- String methods and functions
Module 3: List Object Basics
- Understanding lists and their operations
- List comprehension
- Working with nested lists
Module 4: Tuples, Set, Dictionaries & Its Function
- Tuples: creation, manipulation, unpacking
- Sets: operations, methods
- Dictionaries: key-value pairs, methods, comprehension
Module 5: Function
- Defining and calling functions
- Parameters and arguments
- Return statements and function scope
Module 6: Oops Concepts
- Introduction to Object-Oriented Programming (OOP)
- Classes, objects, attributes, and methods
- Inheritance, encapsulation, polymorphism
Module 7: Files
- Reading from and writing to files
- Working with different file formats such as: text files, CSV, JSON
Module 8: Exception Handling
- Handling errors and exceptions in Python
- try-except blocks, finally clause
Module 9: Memory Management
- Understanding Python’s memory management
- Garbage collection
- Memory optimization techniques
Module 10: Database
- Introduction to database management systems
- Connecting to databases using Python
- CRUD operations: Create, Read, Update, Delete
Module 11: Web API
- Introduction to Web APIs
- Making HTTP requests using Python
- Parsing JSON responses
Module 12: Flask
- Building web applications using Flask framework
- Routing, templates, forms
- RESTful APIs with Flask
Module 13: Pandas Basic
- Introduction to Pandas Library for data manipulation and analysis
- Series, DataFrame, and basic operations
Module 14: Pandas Advance
- Advanced data manipulation with Pandas
- Grouping, filtering, merging, and reshaping data
Module 15: Python Numpy
- Introduction to NumPy library for numerical computing
- Arrays, indexing, slicing, and broadcasting
- Mathematical operations with arrays
Module 16: Visualization
- Data visualization with Matplotlib library
- Plot types: line plots, scatter plots, histograms
- Customizing plots and adding annotations
Each module builds upon the knowledge gained from the previous ones, providing a comprehensive learning experience. Participants will not only learn the fundamentals of Python programming but also gain practical skills in data manipulation, web development, and data visualization.Â
By the end, you’ll have the confidence and expertise to tackle real-world Python projects and pursue lucrative roles in AI and data science.
Read More: & in Python: And Operator in Python
30 Days Of Python For Beginners
Python is a high-level, interpreted programming language known for its simplicity and readability. Unlike low-level languages like C or assembly, Python abstracts away much of the complex syntax, making it easier for beginners to understand and write code quickly.
Here is what you will learn in 30 Days Of Python:
Installing Python
For beginners, installing Python on your system is the first step to start coding. Here’s how you can install Python:
Windows:
- Visit the official Python website at https://www.python.org/.
- Click on the “Downloads” tab.
- Choose the latest version of Python for Windows and download the installer.
- Run the installer and follow the on-screen instructions.
- Make sure to check the option “Add Python to PATH” during installation.
- Once the installation is complete, you can open a command prompt and type Python to verify that Python is installed correctly.
macOS:
- macOS typically comes with Python pre-installed. However, it’s recommended to install the latest version using Homebrew or the official Python installer.
- To install Python using Homebrew, open the Terminal and run the command: brew install python.
- To install Python using the official installer, download it from the Python website and follow the installation instructions.
Linux:
- Most Linux distributions come with Python pre-installed. You can check if Python is installed by opening a terminal and typing Python.
- If Python is not installed or you want to install a different version, you can use your package manager to install it. For example, on Ubuntu, you can use the command: sudo apt-get install python3.
Basic Concepts in Python
- Syntax: Python syntax is clean and easy to read, making it beginner-friendly. It uses indentation to define blocks of code, unlike other languages that use curly braces or keywords.
- Variables: Variables are used to store data values. In Python, you don’t need to declare the data type of a variable explicitly. You can simply assign a value to a variable using the = operator.
# Example of variable assignment
x = 10
name = “Alice”
- Print Statement: The print() function is used to display output to the console.
# Example of print statement
print(“Hello, World!”)
- Comments: Comments are used to explain the code and make it more readable. In Python, comments start with the # symbol.
# This is a comment
print(“Hello, World!”)Â # This is also a comment
- Indentation: Python uses indentation to define blocks of code. Incorrect indentation can lead to syntax errors.
# Example of indentation
if x > 5:
    print(“x is greater than 5”)
else:
    print(“x is less than or equal to 5”)
- Data Types: Python supports various data types such as integers, floats, strings, lists, tuples, sets, dictionaries, etc.
# Example of data types
num = 10Â # Integer
pi = 3.14Â # Float
name = “Alice” # String
- Operators: Python supports various operators for arithmetic, comparison, logical operations, etc.
# Example of operators
result = 10 + 5Â # Addition
is_equal = (10 == 5)Â # Comparison
is_valid = (True and False)Â # Logical
- Input from User: You can take input from the user using the input() function.
# Example of input from user
name = input(“Enter your name: “)
print(“Hello,”, name)
Comparison with Other Languages
- Python vs. C: Python is dynamically typed and has automatic memory management, unlike C which requires explicit memory allocation and deallocation.
- Python vs. Java: Python is more concise and requires fewer lines of code for tasks compared to Java.
- Python vs. JavaScript: Python is often used for server-side scripting and automation, while JavaScript is primarily used for client-side scripting in web development.
How Will 30 Days of Python Learning Help?
Participating in the 30 Days of Python learning program offers several benefits that can help individuals enhance their programming skills and pursue opportunities in various fields such as AI, data science, web development, and more. Here’s how the 30-day Python learning journey can help:
- Structured Learning Path: The program follows a structured curriculum divided into modules, each focusing on specific Python concepts and skills. This organized approach ensures that learners progress steadily from basics to more advanced topics, building a strong foundation along the way.
- Hands-on Practice: Each day of the program includes coding challenges and hands-on projects that allow learners to apply what they’ve learned. This practical experience reinforces understanding and helps solidify programming skills through real-world application.
- Comprehensive Coverage: The curriculum covers a wide range of Python topics, including syntax, data types, control flow, functions, object-oriented programming, file handling, web development, data manipulation, visualization, and more. This comprehensive coverage ensures that learners gain a broad understanding of Python and its applications.
- Portfolio Building: By completing daily challenges and projects, participants will build a portfolio of Python projects that they can showcase to potential employers or use to demonstrate their skills in job interviews. This practical experience adds credibility to their resume and increases their chances of landing lucrative roles in AI, data science, and software engineering.
- Community Support: Learners can interact with peers, ask questions, share insights, and receive guidance from experienced instructors throughout the learning journey, fostering a supportive and collaborative environment.
- Self-paced Learning: The program is designed to accommodate learners with varying schedules and commitments. Participants can progress through the modules at their own pace, allowing flexibility for those with full-time jobs or other responsibilities.
- Career Advancement: Mastering Python opens doors to diverse career opportunities in high-demand fields such as AI, machine learning, data analysis, web development, automation, and more. The skills acquired during the 30 Days of Python program can significantly enhance career prospects and pave the way for professional growth and advancement.
Overall, the 30 Days of Python learning program offers a structured, hands-on approach to mastering Python programming, empowering individuals to acquire valuable skills, build impressive projects, and pursue rewarding career opportunities in the tech industry.
Read More: Python For Data Science: Can Python Be Used In Data Science And Machine Learning?
Python Objects, Numbers & Booleans, Strings
In Python, everything is an object. Objects are instances of data structures or classes, and they can represent data and methods. Let’s explore some basic object types:
- Numbers: Python supports various numerical types such as integers, floats, and complex numbers.
# Examples of numbers
num1 = 10Â # Integer
num2 = 3.14Â # Float
num3 = 2 + 3j # Complex number
- Booleans: Booleans represent truth values, either True or False.
# Examples of booleans
is_python_fun = True
is_learning = False
- Strings: Strings are sequences of characters enclosed within single quotes (”), double quotes (“”) or triple quotes (”’ or “””).
# Examples of strings
name = ‘Alice’
message = “Hello, World!”
multiline_string = ”’This is a
multiline string”’
Container Objects, Mutability Of Objects
Python provides various container objects like lists, tuples, sets, and dictionaries to store collections of data. Container objects can be mutable or immutable:
- Lists: Ordered, mutable collections of objects.
# Example of a list
fruits = [‘apple’, ‘banana’, ‘orange’]
- Tuples: Ordered, immutable collections of objects.
# Example of a tuple
dimensions = (10, 20, 30)
- Sets: Unordered collections of unique objects.
# Example of a set
unique_numbers = {1, 2, 3, 4, 5}
- Dictionaries: Unordered collections of key-value pairs.
# Example of a dictionary
person = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
Python Operators
Operators in Python are symbols that perform operations on operands. They can be arithmetic, comparison, logical, assignment, etc.
# Examples of operators
# Arithmetic operators
result = 10 + 5
# Comparison operators
is_equal = (10 == 5)
# Logical operators
is_valid = (True and False)
# Assignment operators
x = 10
Python Type Conversion
Python allows converting between different data types using type conversion functions like int(), float(), str(), etc.
# Examples of type conversion
# Converting string to integer
num_str = “10”
num_int = int(num_str)
# Converting integer to string
num = 20
num_str = str(num)
- Conditions (If Else, If-Elif-Else)
Conditional statements allow the execution of different code blocks based on specified conditions.
# Example of if-else statement
age = 20
if age >= 18:
    print(“You are an adult”)
else:
    print(“You are a minor”)
- Loops (While, For)
Loops are used to execute a block of code repeatedly. Python supports both while and for loops.
# Example of while loop
count = 0
while count < 5:
    print(“Count:”, count)
    count += 1
# Example of for loop
for i in range(5):
    print(“Number:”, i)
- Break And Continue Statement And Range Function
- Break Statement: Terminates the loop prematurely.
- Continue Statement: Skips the rest of the code inside the loop for the current iteration and proceeds to the next iteration.
- Range Function: Generates a sequence of numbers.
# Example of break statement
for i in range(10):
    if i == 5:
        break
    print(i)
# Example of continue statement
for i in range(10):
    if i == 5:
        continue
    print(i)
# Example of range function
for i in range(1, 10, 2):
    print(i)
Python Namespace
A namespace in Python is a mapping from names to objects. It’s like a dictionary where the keys are the object names and the values are the objects themselves.
# Example of namespace
x = 10
print(“Namespace:”, globals())
Understanding these fundamental concepts and syntax will lay a strong foundation for beginners in Python programming. Each concept will be reinforced through examples and practice exercises throughout the 30 Days Of Python learning journey.
Recommended Technical Course
- Full Stack Development Course
- Generative AI Course
- DSA C++ Course
- Data Analytics Course
- Python DSA Course
- DSA Java Course
30 Days Of Python Cheat Sheet
Here’s a cheat sheet for 30 days of Python, covering various concepts and examples:
Day 1: Introduction to Python
print(“Hello, World!”)
Day 2: Variables and Data Types
# Variables
name = “Alice”
age = 30
# Data Types
string_variable = “Hello”
integer_variable = 42
float_variable = 3.14
boolean_variable = True
Day 3: Operators
# Arithmetic operators
result = 10 + 5
result = 10 – 5
result = 10 * 5
result = 10 / 5
result = 10 % 3
result = 10 ** 2
# Comparison operators
result = 10 > 5
result = 10 < 5
result = 10 == 5
result = 10 != 5
# Logical operators
result = True and False
result = True or False
result = not True
Day 4: Control Flow – if, elif, else
x = 10
if x > 5:
    print(“x is greater than 5”)
elif x < 5:
    print(“x is less than 5”)
else:
    print(“x is equal to 5”)
Day 5: Loops – for loop
for i in range(5):
    print(i)
Day 6: Loops – while loop
i = 0
while i < 5:
    print(i)
    i += 1
Day 7: Lists
my_list = [1, 2, 3, 4, 5]
print(my_list[0])Â # Accessing elements
my_list.append(6)Â # Adding elements
my_list.remove(3)Â # Removing elements
Day 8: Tuples
my_tuple = (1, 2, 3)
print(my_tuple[0])Â # Accessing elements
Day 9: Dictionaries
my_dict = {“name”: “Alice”, “age”: 30}
print(my_dict[“name”])Â # Accessing values
my_dict[“city”] = “New York” # Adding new key-value pair
del my_dict[“age”]Â # Removing a key-value pair
Day 10: Functions
def greet(name):
    print(“Hello,”, name)
greet(“Alice”)
Day 11: Function Arguments
def add(a, b):
    return a + b
result = add(5, 3)
print(result)
Day 12: Function Return Values
def square(x):
    return x ** 2
result = square(4)
print(result)
Day 13: Lambda Functions
square = lambda x: x ** 2
result = square(4)
print(result)
Day 14: Classes and Objects
class Person:
    def __init__(self, name, age):
        self.name = name
        self.age = age
person = Person(“Alice”, 30)
print(person.name)
Day 15: Class Inheritance
class Student(Person):
    def __init__(self, name, age, grade):
        super().__init__(name, age)
        self.grade = grade
student = Student(“Bob”, 25, “A”)
print(student.grade)
Day 16: File Handling
# Writing to a file
with open(“file.txt”, “w”) as file:
    file.write(“Hello, World!”)
# Reading from a file
with open(“file.txt”, “r”) as file:
    content = file.read()
    print(content)
Day 17: Error Handling
try:
    result = 10 / 0
except ZeroDivisionError:
    print(“Cannot divide by zero”)
Day 18: Modules and Packages
# Importing a module
import math
print(math.sqrt(25))
# Creating your own module
# module.py
# def greet(name):
# Â Â print(“Hello,”, name)
#Â
# import module
# module.greet(“Alice”)
Day 19: Virtual Environments
# Create a virtual environment
# Windows
# python -m venv myenv
# Linux/Mac
# python3 -m venv myenv
# Activate virtual environment
# Windows
# myenv\Scripts\activate
# Linux/Mac
# source myenv/bin/activate
Day 20: List Comprehensions
numbers = [1, 2, 3, 4, 5]
squared = [x ** 2 for x in numbers]
print(squared)
Day 21: Set and Dictionary Comprehensions
# Set comprehension
numbers = [1, 2, 3, 4, 5, 5]
unique_numbers = {x for x in numbers}
print(unique_numbers)
# Dictionary comprehension
names = [“Alice”, “Bob”, “Charlie”]
name_lengths = {name: len(name) for name in names}
print(name_lengths)
Day 22: Generators
def countdown(n):
    while n > 0:
        yield n
        n -= 1
for i in countdown(5):
    print(i)
Day 23: Decorators
def my_decorator(func):
    def wrapper():
        print(“Something is happening before the function is called.”)
        func()
        print(“Something is happening after the function is called.”)
    return wrapper
@my_decorator
def say_hello():
    print(“Hello!”)
say_hello()
Day 24: Map, Filter, and Reduce
# Map
numbers = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x ** 2, numbers))
print(squared)
# Filter
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers)
# Reduce
from functools import reduce
sum_of_numbers = reduce(lambda x, y: x + y, numbers)
print(sum_of_numbers)
Day 25: Regular Expressions
import re
text = “The rain in Spain”
matches = re.findall(“ai”, text)
print(matches)
Day 26: JSON
import json
# Encode Python object to JSON
person = {“name”: “Alice”, “age”: 30}
json_string = json.dumps(person)
print(json_string)
# Decode JSON to Python object
person = json.loads(json_string)
print(person)
Day 27: Date and Time
import datetime
# Current date and time
current_date = datetime.datetime.now()
print(current_date)
# Format date and time
formatted_date = current_date.strftime(“%Y-%m-%d %H:%M:%S”)
print(formatted_date)
# Create a specific date
specific_date = datetime.datetime(2023, 12, 25)
print(specific_date)
Day 28: Math and Random
import math
import random
# Math
print(math.sqrt(25))
# Random
print(random.randint(1, 10))
Day 29: Sending Email
import smtplib
from email.mime.text import MIMEText
msg = MIMEText(“Hello, this is a test email.”)
msg[“Subject”] = “Test”
msg[“From”] = “sender@example.com”
msg[“To”] = “recipient@example.com”
server = smtplib.SMTP(“smtp.example.com”, 587)
server.starttls()
server.login(“username”, “password”)
server.sendmail(“sender@example.com”, “recipient@example.com”, msg.as_string())
server.quit()
Day 30: Web Scraping (using BeautifulSoup)
from bs4 import BeautifulSoup
import requests
url = “https://example.com”
response = requests.get(url)
soup = BeautifulSoup(response.text, “html.parser”)
# Extracting data
title = soup.title.string
print(“Title:”, title)
# Finding all links
links = soup.find_all(“a”)
for link in links:
    print(link.get(“href”))
Through this is comprehensive 30-day of Python journey, you will gain a solid grasp of Python’s fundamentals and practical abilities to tackle real-world projects. From mastering syntax, data structures, and control flows to advanced concepts like OOP, web scraping, and more – this cheat sheet summarizes the rewarding path ahead.
In the PW Skills’ DSA Python course with each concept reinforced by examples and exercises, you will code alongside expert instructors each step of the way. By the end, you will have the portfolio and modern skillset needed to pursue lucrative roles in AI, data science, and software engineering.
We highly recommend enrolling in this thoughtfully structured course. Whether you’re a beginner looking to break into coding or a professional aiming to level up your abilities, the 30 Days of Python program delivers an illuminating and engaging learning experience. The time to unlock your potential is now – reserve your seat and let this comprehensive guide elevate your skills.
For Latest Tech Related Information, Join Our Official Free Telegram Group : PW Skills Telegram Group
30-day of Python FAQs
Is prior programming experience required to participate in the 30 Days of Python course?
No, the course is designed for beginners with no prior programming experience. The curriculum starts with the basics of Python programming and gradually progresses to more advanced topics, making it accessible to learners of all levels.
Can I enroll in the 30 Days of Python program if I have a full-time job or other commitments?
Absolutely! The course is self-paced, allowing you to study and complete coding challenges at your convenience. Daily challenges are designed to be beginner-friendly and can typically be completed within a reasonable amount of time each day.
Will I receive any support or assistance during the 30 Days of Python journey?
Yes, PW Skills provides comprehensive support throughout the course. This includes access to community forums where you can interact with fellow learners and instructors, as well as supplementary materials such as notes, exercises, and mentorship to enhance your learning experience.
What kind of projects will I build during the 30 Days of Python program?
Throughout the course, you will work on a variety of real-world projects that reinforce the concepts covered in each module. These projects range from basic applications like a text-based game or a to-do list manager to more complex tasks such as web scraping or data visualization.
Is there a fee to enroll in the 30 Days of Python course?
No, the course is free to enroll. PW Skills believes in making quality education accessible to everyone, so there are no upfront costs associated with joining the program. Simply reserve your seat and start your journey towards mastering Python for AI and data science applications.