1 Python represents an integer value. Integers are whole numbers that can be positive, negative, or zero. 1 is the first and smallest positive integer. Integers are immutable in Python, meaning their value can’t be changed once assigned. 1 is commonly used to initialize values, counters, and arrays. It is also used in Boolean expressions evaluating to True.
1 Python: In the Python programming language, 1 is an integer literal representing a whole number value. Integers are immutable, meaning their values cannot be changed once assigned. The integer 1 has several important uses in Python code that you will learn in this guide.Â
So, while seemingly basic on the surface, the integer 1 plays a major role in Python coding. Mastering numeric data types like 1, floats, and complex numbers is critical for both beginning Python programmers and experienced developers.Â
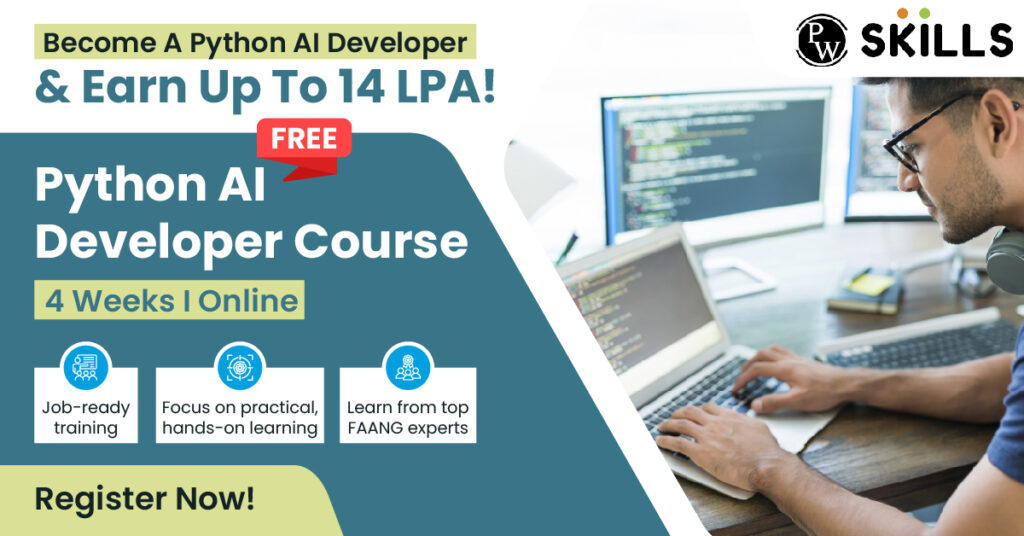
Our comprehensive Python For AI Course at PW Skills teaches integers and other core Python concepts like strings, lists, conditionals, functions, object-oriented programming, web development with Flask, and more.Â
The course contains quizzes, exercises, and projects to help cement your Python skills. Enroll today and learn how to leverage the power of 1 and other Python fundamentals.
Define :- 1 Python
In Python, -1 is an integer literal representing the negative integer value of one. It is essentially the opposite of 1 and falls within the category of integer data types. Like other integers, -1 is immutable, meaning its value cannot be changed once assigned.
Here’s a detailed breakdown of -1 in Python:
Integer Literal: -1 is a literal in Python representing a negative integer value.
Significance: -1 holds significance in various computational scenarios where negative numbers are required. It is commonly used in mathematical calculations, algorithms, and logical operations.
Representation: -1 is represented in binary, octal, and hexadecimal formats just like any other integer. For instance:
- Binary: -1 is 0b11111111.
- Octal: -1 is 0o377.
- Hexadecimal: -1 is 0xff.
Usage:
- Arithmetic Operations: -1 can be used in arithmetic operations like addition, subtraction, multiplication, and division.
- Conditional Statements: -1 can be used in conditional statements such as if, elif, and else.
- Iterative Constructs: -1 can be used as loop counters or as the stopping criteria in iterative constructs like for loops and while loops.
Example:
# Using -1 in arithmetic operations
result = -1 + 5
print(“Result of -1 + 5 =”, result)Â # Output: Result of -1 + 5 = 4
# Using -1 in conditional statements
num = -1
if num < 0:
    print(“Number is negative”)
elif num == 0:
    print(“Number is zero”)
else:
    print(“Number is positive”)
# Output: Number is negative
# Using -1 in iterative constructs
for i in range(-1, 2):
    print(i, end=’ ‘)
# Output:Â
-1 0 1
In the above example, -1 is used in arithmetic operations, conditional statements, and iterative constructs to demonstrate its versatility and usage in various contexts within Python programming.
Read More: Python Tutorial | Learn Python Programming
Understanding Different Representations of 1 Python
In Python, the value 1 can be represented in various ways, offering flexibility and versatility in coding. Let’s explore two common methods:
- Using Square Brackets with No Colon:
Syntax:Â
[SEQUENCE[-1]]
Explanation:
- Here, the square brackets indicate indexing or accessing elements within a sequence.
- The -1 inside the brackets represents accessing the last element of the sequence.
- This method is straightforward and useful when you want to retrieve the last item without any additional complexity.
- Using Double Colon Syntax:
Syntax:Â
[SEQUENCE[::-1]]
Explanation:
- In this syntax, the double colon (::) signifies a slicing operation on the sequence.
- The -1 after the second colon indicates that the sequence should be traversed in reverse.
- This approach is handy when you need to reverse the entire sequence, such as a string, list, or dictionary.
- General Syntax for Slicing Operations:
Syntax:Â
SEQUENCE[START: STOP: STEP]
Explanation:
- The START parameter denotes the starting index number.
- The STOP parameter indicates the end or last index number.
- The optional STEP parameter provides an increment for selection, with -1 initializing a reverse traversal.
Sequences can include strings, lists, or dictionaries, offering flexibility in application.
Understanding these different representations of 1 Python allows for efficient manipulation and access of sequence elements, contributing to clearer and more concise code.
Python [::-1] Reverse
In Python, the expression [::-1] is used to reverse a sequence. This sequence can be a string, list, tuple, or any other iterable object. Let’s delve into the details of this expression along with examples to illustrate its usage:
- Syntax Explanation:
- [::-1]: This slicing syntax consists of three parts:
- start: Indicates the starting index of the slice (default is the beginning).
- stop: Indicates the stopping index of the slice (default is the end).
- step: Indicates the step size (default is 1). When -1 is used, it traverses the sequence in reverse.
- So, [start:stop:step] with start and stop omitted and step as -1 results in reversing the sequence.
- Usage:
- Reversing a Sequence: The primary purpose of [::-1] is to reverse the elements of a sequence.
- Useful for Palindrome Checking: This syntax is commonly used to check if a sequence is a palindrome, as comparing the original sequence with its reversed version can determine if it reads the same forwards and backward.
Example:
Let’s demonstrate the usage of [::-1] with different types of sequences:
String Reversal:
original_string = “hello”
reversed_string = original_string[::-1]
print(“Original string:”, original_string)
print(“Reversed string:”, reversed_string)
# Output: Original string: hello
# Â Â Â Â Reversed string: olleh
List Reversal:
original_list = [1, 2, 3, 4, 5]
reversed_list = original_list[::-1]
print(“Original list:”, original_list)
print(“Reversed list:”, reversed_list)
# Output: Original list: [1, 2, 3, 4, 5]
# Â Â Â Â Reversed list: [5, 4, 3, 2, 1]
Tuple Reversal:
original_tuple = (10, 20, 30, 40, 50)
reversed_tuple = original_tuple[::-1]
print(“Original tuple:”, original_tuple)
print(“Reversed tuple:”, reversed_tuple)
# Output: Original tuple: (10, 20, 30, 40, 50)
# Â Â Â Â Reversed tuple: (50, 40, 30, 20, 10)
Palindrome Checking Example:
def is_palindrome(s):
    return s == s[::-1]
string1 = “radar”
string2 = “hello”
print(string1, “is palindrome?”, is_palindrome(string1))Â # Output: radar is palindrome? True
print(string2, “is palindrome?”, is_palindrome(string2))Â # Output: hello is palindrome? False
In summary, [::-1] is a concise and powerful way to reverse a sequence in Python, making it a handy tool for various tasks such as string manipulation, list operations, and palindrome checking.
S[::-1] Meaning In Python
In Python, the expression s[::-1] is a slicing operation used to reverse a sequence s, where s can be a string, list, tuple, or any other iterable object. Let’s explore this expression in detail along with examples to illustrate its functionality:
- Syntax Explanation:
- s[::-1]: This slicing syntax consists of three parts:
- start: Indicates the starting index of the slice (default is the beginning).
- stop: Indicates the stopping index of the slice (default is the end).
- step: Indicates the step size (default is 1). When -1 is used as the step, it traverses the sequence in reverse.
So, [start:stop:step] with start and stop omitted and step as -1 results in reversing the sequence.
- Usage:
- Reversing a Sequence: The primary purpose of s[::-1] is to reverse the elements of the sequence s.
- No Modification to Original: It’s important to note that s[::-1] does not modify the original sequence s; rather, it creates a new reversed sequence.
Example:
Let’s demonstrate the usage of s[::-1] with different types of sequences:
String Reversal:
original_string = “hello”
reversed_string = original_string[::-1]
print(“Original string:”, original_string)
print(“Reversed string:”, reversed_string)
# Output: Original string: hello
# Â Â Â Â Reversed string: olleh
List Reversal:
original_list = [1, 2, 3, 4, 5]
reversed_list = original_list[::-1]
print(“Original list:”, original_list)
print(“Reversed list:”, reversed_list)
# Output: Original list: [1, 2, 3, 4, 5]
# Â Â Â Â Reversed list: [5, 4, 3, 2, 1]
Tuple Reversal:
original_tuple = (10, 20, 30, 40, 50)
reversed_tuple = original_tuple[::-1]
print(“Original tuple:”, original_tuple)
print(“Reversed tuple:”, reversed_tuple)
# Output: Original tuple: (10, 20, 30, 40, 50)
# Â Â Â Â Reversed tuple: (50, 40, 30, 20, 10)
Advantages:
Concise and Readable: s[::-1] provides a concise and readable way to reverse a sequence without needing to write explicit loops or functions.
Immutable Sequences: This approach works well with immutable sequences like strings and tuples, where the original sequence cannot be modified.
In summary, s[::-1] is a powerful and elegant way to reverse a sequence in Python, offering simplicity, readability, and versatility in various programming scenarios.
Read More: Backend In Python: 6 Reasons To Choose Python For Backend Development
Exploring the Difference Between a[-1] and a[::-1] in Python
In Python, a[-1] and a[::-1] both involve accessing elements of a sequence, such as a list or a string, but they have different functionalities.
a[-1]:
- This notation retrieves the last element of the sequence a.
- It accesses the element at index -1, which is the last element in the sequence.
- It’s useful when you specifically want to access the last element without reversing the entire sequence.
Example:
my_list = [10, 20, 30, 40, 50]
last_element = my_list[-1]
print(“Last Element:”, last_element)Â
# Output: Last Element: 50
a[::-1]:
- This notation reverses the entire sequence a.
- It uses slicing syntax [start:stop:step], where start and stop are omitted (defaulting to the beginning and end of the sequence, respectively), and step is -1, indicating that the sequence should be traversed in reverse.
- It’s useful when you want to reverse the entire sequence.
Example:
my_string = “hello”
reversed_string = my_string[::-1]
print(“Reversed String:”, reversed_string)Â Â
# Output: Reversed String: olleh
In summary, while a[-1] retrieves the last element of the sequence, a[::-1] reverses the entire sequence. They serve different purposes and are used accordingly based on the desired outcome in Python programming.
L(1 Python)
L(1 Python) or L1 regularization, also known as Lasso regularization, is a technique commonly used in machine learning to prevent overfitting and select important features in a model. It adds a penalty term to the loss function that is proportional to the absolute value of the coefficients. This penalty encourages the coefficients to be small, effectively driving some of them to zero, thereby performing feature selection.
Here’s a detailed breakdown of how to implement L1 regularization in Python using the scikit-learn library:
Import Necessary Libraries:
First, you need to import the Lasso class from the linear_model module in scikit-learn:
from sklearn.linear_model import Lasso
Create Lasso Model:
Initialize a Lasso regression model by specifying the value of the regularization parameter alpha. A smaller alpha value leads to weaker regularization, while a larger value leads to stronger regularization:
lasso = Lasso(alpha=0.1)
Fit the Model:
Fit the Lasso model to your training data (X and y), where X represents the features and y represents the target variable:
lasso.fit(X, y)
Make Predictions:
Once the model is trained, you can use it to make predictions on new data (X_new):
predictions = lasso.predict(X_new)
Example:
Here’s a complete example demonstrating how to use L1 regularization (Lasso) with scikit-learn for linear regression:
from sklearn.linear_model import Lasso
import numpy as np
# Generate some synthetic data
np.random.seed(0)
X = 2 * np.random.rand(100, 3)
y = 5 + np.dot(X, np.array([1, 2, 3])) + np.random.randn(100)
# Create a Lasso model
lasso = Lasso(alpha=0.1)
# Fit the model to the data
lasso.fit(X, y)
# Make predictions
X_new = np.array([[0.5, 0.5, 0.5], [1, 1, 1]])
predictions = lasso.predict(X_new)
print(“Coefficients:”, lasso.coef_)
print(“Intercept:”, lasso.intercept_)
print(“Predictions:”, predictions)
In this example, we generate synthetic data, create a Lasso model with alpha=0.1, fit it to the data, and make predictions on new data points (X_new). Finally, we print the coefficients, intercept, and predictions.
Recommended Technical CourseÂ
1 Python Download
Downloading Python typically involves getting the Python interpreter, which is the core software that allows you to run Python code. Here are the steps to download Python, along with an example:
Visit the Official Python Website:
Go to the official Python website, which is https://www.python.org/. On the homepage, you will find a “Downloads” tab. Click on it.
Select the Python Version:
Choose the Python version that suits your needs. There are typically two versions available – the latest stable version and the latest version in the pre-release stage. For most users, the latest stable version is recommended.
Choose the Operating System:
Select your operating system. Python is compatible with Windows, macOS, and various Linux distributions. Make sure to choose the correct version for your operating system.
Download the Installer:
Click on the download link to get the installer for your chosen version and operating system. The installer includes the Python interpreter, standard libraries, and additional tools.
Run the Installer:
Once the installer is downloaded, run it to start the installation process. Follow the installation wizard, and make sure to check the option that adds Python to your system’s PATH. This makes it easier to run Python from the command line.
Verify the Installation:
After the installation is complete, open a command prompt or terminal and type python –version or python -V to check the installed Python version. You should see the version number you downloaded.
Example:
Let’s consider an example for downloading and installing Python on a Windows system.
- Visit https://www.python.org/.
- Click on “Downloads.”
- Choose the latest stable version for Windows.
- Download the Windows installer (e.g., Python 3.9.7).
- Run the installer, ensuring the option to add Python to PATH is selected.
- Open a command prompt and type python –version.
C:\Users\YourUsername>python –version
Python 3.9.7
This indicates that Python 3.9.7 is successfully installed on your system.
Downloading Python is a straightforward process, and it allows you to start coding in Python on your machine. Once installed, you can use Python for various tasks, including scripting, web development, data analysis, and more.
In conclusion, Python is a versatile language with various applications in programming, data science, and machine learning. From understanding basic integer literals like 1 to utilizing advanced techniques like L1 regularization, Python offers a wide range of tools for developers at every level.Â
Whether you’re a beginner or an experienced programmer, mastering Python fundamentals is crucial. Explore our comprehensive Python For AI Course at PW Skills, designed to deepen your understanding of core concepts while offering practical exercises and projects.Â
Enroll today to unlock the power of Python and advance your skills in AI development.
For Latest Tech Related Information, Join Our Official Free Telegram Group : PW Skills Telegram Group
1 Python FAQs
Why is the integer 1 significant in Python programming?
The integer 1 in Python holds significance as the smallest positive whole number. It is commonly used for initializing values, counters, and arrays. Additionally, it plays a crucial role in Boolean expressions, often evaluating to True.
How is the L1 regularization (Lasso) applied in machine learning using Python?
L1 regularization, also known as Lasso regularization, is implemented in Python using the scikit-learn library. The process involves importing the Lasso class, creating a Lasso model with a specified alpha value, fitting the model to training data, and making predictions on new data.
What is the purpose of the slicing expression [::-1] in Python?
The slicing expression [::-1] is used to reverse a sequence in Python. Whether it's a string, list, tuple, or any iterable object, [::-1] effectively reverses the elements. It is often employed in tasks such as string manipulation, list operations, and palindrome checking.
How does Python [-1] differ from Python [::-1]?
Python[-1] retrieves the last element of a sequence, while Python[::-1] reverses the entire sequence. The former is useful when accessing the last element specifically, while the latter is employed to reverse the entire sequence.
Why should one consider enrolling in the Python For AI Course at PW Skills?
The Python For AI Course at PW Skills offers a comprehensive learning experience, covering fundamental concepts such as integers, strings, lists, conditionals, functions, and more. It provides practical exercises, quizzes, and projects to strengthen Python skills, making it beneficial for both beginners and experienced developers aiming to delve into AI development.